Basic PHP Functions for Novice Users – Part 2
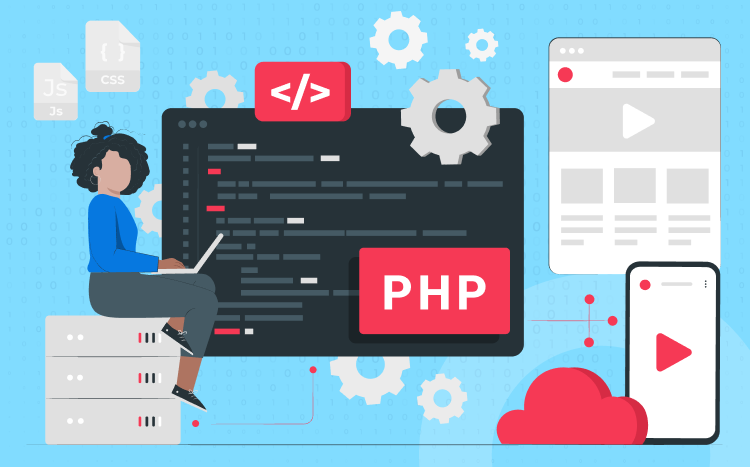
Welcome back to our blog series on basic PHP functions for novice users of Tierra Hosting! In our previous post, we covered some essential PHP functions, including file uploading and MySQL database connection. In this post, we will explore a few more commonly used PHP functions that can help you enhance your website's functionality.
The mail() Function:
If you want to send emails from your PHP code, you can use the mail() function. This function allows you to send emails with specified recipients, subject, message, headers, and additional parameters. Here's an example:
<?php $to = "recipient@example.com"; $subject = "Hello"; $message = "This is a test email sent from PHP."; $headers = "From: sender@example.com\r\n" . "Reply-To: sender@example.com\r\n" . "X-Mailer: PHP/" . phpversion(); if (mail($to, $subject, $message, $headers)) { echo "Email sent successfully."; } else { echo "Failed to send email."; } ?>
The date() Function:
The date() function in PHP allows you to retrieve the current date and time or format a given timestamp into a human-readable date string. You can use various format codes to customize the output. Here's an example:<?php echo "Today is " . date("Y-m-d") . ""; echo "The time is " . date("h:i:sa") . ""; echo "Today is " . date("l") . ""; ?>
The filter_var() Function:
The filter_var() function in PHP is used for data validation and filtering. It allows you to validate and sanitize various types of data, such as email addresses, URLs, integers, and more. Here's an example:<?php $email = "user@example.com"; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo "$email is a valid email address."; } else { echo "$email is not a valid email address."; } ?>
Conclusion:
These are a few more examples of basic PHP functions that can be useful for novice users of Tierra Hosting. PHP provides a wide range of functions to perform various tasks, from handling emails to validating user input and formatting date and time. Understanding and using these functions can help you enhance your website's functionality and provide a better user experience. We hope you find this continuation helpful in your PHP learning journey!
If you reached this page directly from a link, be sure to start over at the first post of this series, "Basic PHP Functions for Novice Users - Part 1".