Basic PHP Functions for Novice Users
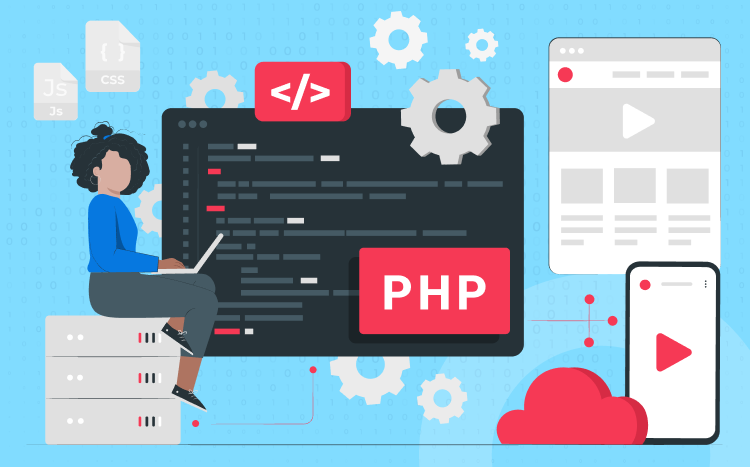
If you're new to web hosting and PHP programming, here are some basic PHP functions that you can use to perform common tasks on your website hosted with Tierra Hosting.
The header() Function:
The header() function in PHP allows you to send HTTP headers to the browser, which can be used for various purposes, such as redirecting users to another page or setting response headers. Here's an example:<?php // Redirect to another page after 5 seconds header("refresh:5;url=another_page.php"); echo "You will be redirected to another page in 5 seconds..."; ?>
The mail() Function:
If you want to send an email from your website, you can use the mail() function in PHP. This function allows you to specify the recipient email address, subject, and message, among other options. Here's a basic example:<?php $to = "you@example.com"; $subject = "Hello from Tierra Hosting"; $message = "This is a test email sent from PHP."; $headers = "From: webmaster@example.com" . "\r\n" . "CC: another@example.com"; mail($to, $subject, $message, $headers); ?>
The move_uploaded_file() Function:
If you want to handle file uploads on your website, you can use the move_uploaded_file() function in PHP. This function allows you to move an uploaded file to a new location on the server. Here's an example:<?php $target_dir = "uploads/"; $target_file = $target_dir . basename($_FILES["file"]["name"]); $uploadOk = 1; $imageFileType = strtolower(pathinfo($target_file,PATHINFO_EXTENSION)); // Check if image file is a actual image or fake image if(isset($_POST["submit"])) { $check = getimagesize($_FILES["file"]["tmp_name"]); if($check !== false) { echo "File is an image - " . $check["mime"] . "."; $uploadOk = 1; } else { echo "File is not an image."; $uploadOk = 0; } } // Check if file already exists if (file_exists($target_file)) { echo "Sorry, file already exists."; $uploadOk = 0; } // Check file size if ($_FILES["file"]["size"] --> 500000) { echo "Sorry, your file is too large."; $uploadOk = 0; } // Allow certain file formats if($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg" && $imageFileType != "gif" ) { echo "Sorry, only JPG, JPEG, PNG & GIF files are allowed."; $uploadOk = 0; } // Check if $uploadOk is set to 0 by an error if ($uploadOk == 0) { echo "Sorry, your file was not uploaded."; // If everything is ok, try to upload file } else { if (move_uploaded_file($_FILES["file"]["tmp_name"], $target_file)) { echo "The file ". htmlspecialchars( basename( $_FILES["file"]["name"])). " has been uploaded."; } else { echo "Sorry, there was an error uploading your file."; } } ?>
The mysqli_connect() Function:
If you want to connect to a MySQL database from your PHP code, you can use the mysqli_connect() function. This function allows you to establish a connection to the database server and perform database operations, such as inserting, updating, and retrieving data. Here's an example:<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database_name"; // Create connection $conn = mysqli_connect($servername, $username, $password, $dbname); // Check connection if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } // Perform database operations here // Close connection mysqli_close($conn); ?>
Please note that you would need to replace the placeholder values (e.g., username, password, database_name, etc.) in the MySQLi example with your actual database credentials in order for it to work correctly.