Securing Your PHP Code: Best Practices
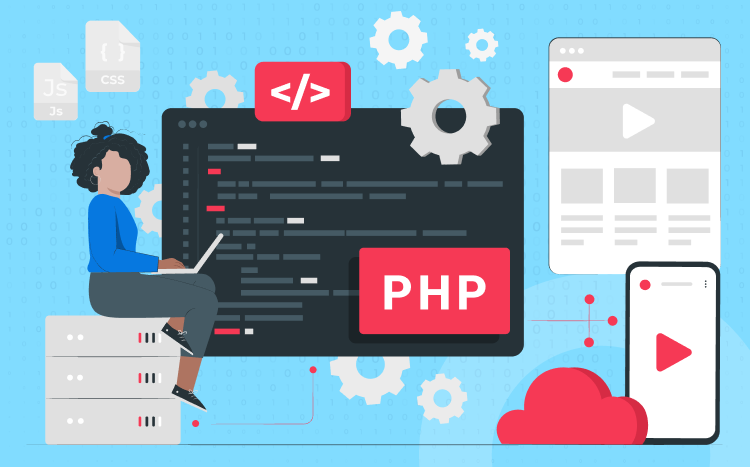
In this post, we will explore some best practices for securing your PHP code and protecting your web applications from common security threats. PHP has built-in features and functions that can help you write secure code and minimize the risk of vulnerabilities.
Securing your PHP code is crucial to protect your web applications from potential security threats. Here are some best practices you can follow:
-
Use Prepared Statements: Prepared statements are a technique used to prevent SQL injection attacks, which are one of the most common forms of attacks against PHP applications. Prepared statements use placeholders to separate SQL queries from user input, preventing malicious SQL code from being executed. For example:
<?php // Using prepared statements with PDO $stmt = $pdo->prepare("SELECT * FROM users WHERE username = ?"); $stmt->execute([$username]); $user = $stmt->fetch(); ?>
-
Validate and Sanitize User Input: User input can be a potential entry point for attacks such as cross-site scripting (XSS) and cross-site request forgery (CSRF). It's important to validate and sanitize all user input to ensure it meets expected formats and does not contain malicious code. For example:
<?php $username = $_POST['username']; $password = $_POST['password']; // Validate and sanitize user input if (filter_var($username, FILTER_VALIDATE_EMAIL)) { // Valid email address } else { // Invalid email address } $username = htmlspecialchars($username); $password = md5($password); ?>
-
Enable Error Reporting and Logging: Error reporting and logging are essential for identifying and fixing security issues in your PHP code. Enabling error reporting in your development environment and logging errors to a secure location can help you quickly detect and resolve potential vulnerabilities. For example:
<?php // Enable error reporting error_reporting(E_ALL); ini_set('display_errors', 1); // Log errors to a file ini_set('log_errors', 1); ini_set('error_log', '/path/to/error.log'); ?>
-
Use Secure Authentication and Authorization: Proper authentication and authorization mechanisms are crucial for protecting user accounts and data. Avoid storing passwords in plaintext and use secure hashing algorithms, such as bcrypt, for storing password hashes. Implement role-based access control (RBAC) to ensure users only have access to the resources they are authorized for. For example:
<?php // Storing password hashes using bcrypt $password = "mypassword"; $hash = password_hash($password, PASSWORD_BCRYPT); // Verifying password against stored hash if (password_verify($password, $hash)) { // Password is correct } else { // Password is incorrect } ?>
-
Keep PHP and Libraries Up to Date: Regularly updating PHP and its libraries is crucial for staying protected against known security vulnerabilities. Stay updated with the latest releases and security patches for PHP and any third-party libraries that you use in your code. Outdated versions of PHP and libraries may have known security vulnerabilities that can be exploited by attackers. Keep an eye on security advisories and updates from PHP and library developers, and apply patches or updates as soon as they are released. For example:
<?php // Update PHP to the latest stable version // Check for updates from PHP's official website or repository // Update third-party libraries to the latest versions // Check for updates from library developers or official documentation ?>
By keeping PHP and its libraries up to date, you can ensure that your code is protected against known security vulnerabilities and reduce the risk of potential attacks. Regular updates help in maintaining the security and stability of your PHP application.